React에서 이벤트 처리 정리 및 예제 - onClick, bind, setState
# 리액트(React)의 이벤트 문법
리액트에서는 함수명을 카멜케이스로 작성하고 onclick(전부 소문자)으로 사용하는 자바스크립트와 달리 onClick 으로 사용합니다.
# 이벤트 기본 동작 방지
<a href="#" onClick={clickEventFunc}>
클릭하세요
</a>
<a> 태그에 onClick 이벤트를 설정하면 기본적으로 href로 페이지를 이동하는 기본 동작이 있습니다.
e.preventDefault();
를 사용하여 이를 동작하지 않게 하고 onClick에 넘겨준 것만 실행할 수 있게 해줍니다.
function clickEventFunc(e){
e.preventDefault();
console.log('페이지 이동 없이 클릭');
}
event를 인자로 받아 e.preventDefault()
를 호출하면, 이벤트의 기본동작은 동작하지 않게 되고 console.log
만 수행됩니다.
# function 바인딩 - bind()
기본적으로 onClick
등으로 전달한 이벤트에서 this
를 호출한 경우 바인딩 되어있지 않기 때문에 undefined
로 표시됩니다.
this는 render()함수가 포함되어 있는 컴포넌트 자체를 가르키는데 그 안에있는 function 내부에서 this는 바인딩을 해주지 않으면 아무값도 가지지 않습니다. 이러한 이유로 this를 바인딩 해 주어야 합니다.
아래와 같이 바인딩을 해주지 않으면 this의 state를 바꾸기 위해 this.setState
를 하는데 undefined
오류가 발생합니다.
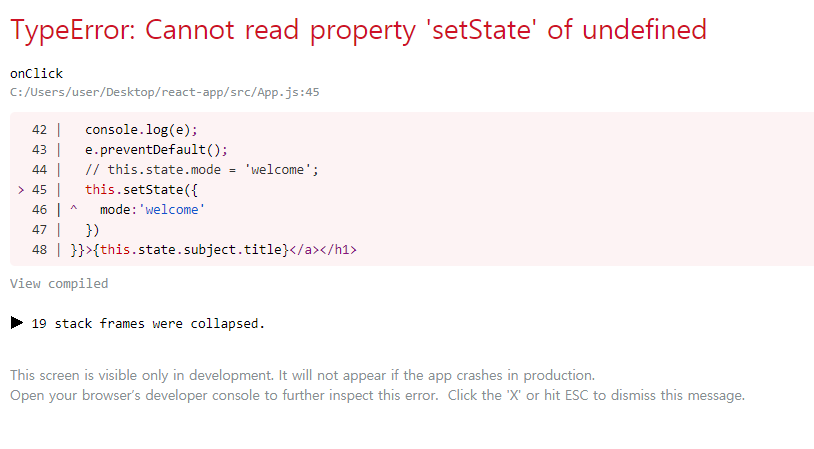
아래와 같이 해당 function
에 .bind(this)
로 this를 바인딩 시켜줍니다.
<h1>
<a href="/" onClick={function(e){
console.log(e);
e.preventDefault();
// this.state.mode = 'welcome';
this.setState({
mode:'welcome'
})
}.bind(this)}>{this.state.subject.title}</a> // 해당 function에 .bind(this)로 this를 바인딩 시켜준다.
</h1>
React 리액트 컴포넌트 이벤트 처리 기본예제 1
아래 예제는 이해를 돕기 위해 function을 따로 빼지 않고 onClick={function(e){...}.bind(this)} 로 직접 처리하였습니다.
App.js
- 부모 컴포넌트
import React, { Component } from 'react';
import TOC from "./components/TOC"
import Content from "./components/Content"
import Subject from "./components/Subject"
import './App.css';
class App extends Component{
// 컴포넌트가 실행될 때 constructor가 먼저 실행되면서 초기화를 담당한다.
constructor(props){
super(props);
this.state ={
mode:'read',
subject:{title:'WEB', sub:"World Wide Web!"},
welcome:{title:'Welcome', desc:'Hello, React!!'},
contents:[
{id:1, title:'HTML', desc:"HTML is for information"},
{id:2, title:'CSS', desc:"CSS is for design"},
{id:3, title:'JavaScript', desc:"JavaScript is for interactive"}
]
}
}
render(){ // 렌더링이 될 때마다 state의 mode값을 확인하여 이벤트 처리
console.log('App render');
var _title, _desc = null;
if(this.state.mode === 'welcome'){
_title = this.state.welcome.title;
_desc = this.state.welcome.desc;
}else if(this.state.mode === 'read'){
_title = this.state.contents[0].title;
_desc = this.state.contents[0].desc;
}
return (
<div className="App">
{/* <Subject
title={this.state.subject.title}
sub={this.state.subject.sub}>
</Subject> */}
<header>
<h1><a href="/" onClick={function(e){
console.log(e);
e.preventDefault(); // a 태그의 기본 동작은 href로 페이지 이동이기 때문에 이동을 막기 위해 사용
// this.state.mode = 'welcome'; // 이렇게 하면 react는 state가 바꼈다는걸 인식하지 못하기 때문에 setState를 사용합니다.
this.setState({ // 클릭 시 state의 mode의 값을 'welcome'으로 바꿉니다.
mode:'welcome'
})
}.bind(this)}>{this.state.subject.title}</a></h1> // function에서 this는 바인딩되어있지 않기 때문에 bind(this)를 통해 this를 바인딩
{this.state.subject.sub}
</header>
<TOC data={this.state.contents}></TOC>
<Content title={_title} desc={_desc}></Content> // 렌더링 될 때 정의된 값을 넣어줍니다.
</div>
);
}
}
export default App;
Content.js
- 자식 컴포넌트
import React, { Component } from 'react';
class Content extends Component{
render(){
console.log('Content render');
return(
<article>
<h2>{this.props.title}</h2>
{this.props.desc}
</article>
);
}
}
export default Content;
해서 이벤트에 따라 props의 던지는 값이 다르기 때문에 Content 컴포넌트도 이벤트에 따라 렌더링이 다시 되면서 props 값에 따라 화면을 다시 그리게 됩니다.
브라우저 화면
[WEB] 버튼 클릭 전
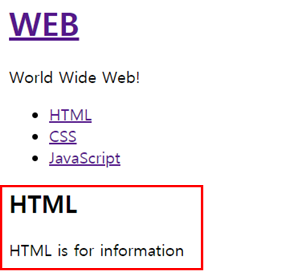
[WEB] 버튼 클릭 후
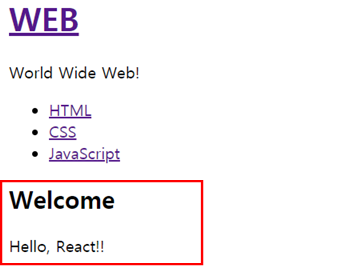
React 리액트 컴포넌트 이벤트 처리 기본예제 2
이번에는 자식 컴포넌트를 직접 호출하여 사용할 때 이벤트 처리 예제입니다.
App.js
- 부모 컴포넌트
return (
<div className="App">
<Subject
title={this.state.subject.title}
sub={this.state.subject.sub}
// 이벤트 함수 작성
onChangePage = {function(){
this.setState({mode:'welcome'});
}.bind(this)}
>
</Subject>
<TOC data={this.state.contents}></TOC>
<Content title={_title} desc={_desc}></Content>
</div>
);
Subject 안에 onChangePage
라는 이벤트를 작성하고 자식 컴포넌트 쪽에서 이벤트를 호출 하는 형식으로 코드를 작성합니다. 이제 컴포넌트 사용자가 설치한 onChagePage 함수를 호출해 주기만 하면 됩니다.
onChangePage
함수는 어떠한 형태로 Subject.js
에 전달될까요? 바로 props
로 전달되겠죠?
Subject.js
- 자식 컴포넌트
import React, { Component } from 'react';
class Subject extends Component{
render(){
console.log('Subject render');
return(
<header>
<h1><a href="/" onClick={function(e){ // a 태그가 onClick될 때
e.preventDefault(); // a태그의 기본 작동인 href 이동 막음
this.props.onChangePage(); // props로 받아온 onChagePage()함수 호출
}.bind(this)}>{this.props.title}</a></h1>
{this.props.sub}
</header>
);
}
}
export default Subject;
브라우저 화면
[WEB] 버튼 클릭 전
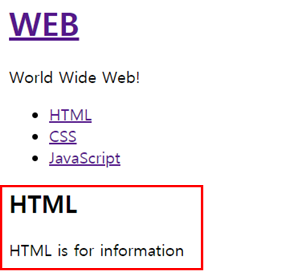
[WEB] 버튼 클릭 후
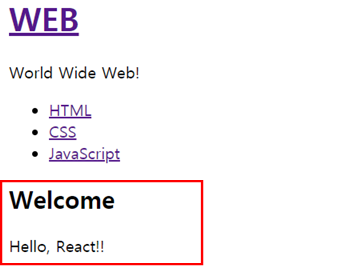
리액트 글 모두 보러 가기
devmoony.tistory.com/category/Web/React%20%EB%A6%AC%EC%95%A1%ED%8A%B8
'Web/React 리액트' 카테고리의 글 목록
devmoony.tistory.com
'Programming > React' 카테고리의 다른 글
[React 리액트] setState() - state 값 동적 변경 (0) | 2021.04.09 |
---|---|
[React 리액트] bind() 함수 이해하기 (개념 및 사용법) (0) | 2021.04.08 |
[React 리액트] Each child in a list shoul have a unipue "key" prop 오류 해결 방법 (0) | 2021.04.07 |
[React 리액트] 리스트(배열) 데이터 여러 개 출력하는 방법 (props, key) (0) | 2021.04.01 |
[React 리액트] props와 state 개념 및 기본 예제 (0) | 2021.03.31 |
React에서 이벤트 처리 정리 및 예제 - onClick, bind, setState
# 리액트(React)의 이벤트 문법
리액트에서는 함수명을 카멜케이스로 작성하고 onclick(전부 소문자)으로 사용하는 자바스크립트와 달리 onClick 으로 사용합니다.
# 이벤트 기본 동작 방지
<a href="#" onClick={clickEventFunc}> 클릭하세요 </a>
<a> 태그에 onClick 이벤트를 설정하면 기본적으로 href로 페이지를 이동하는 기본 동작이 있습니다.
e.preventDefault();
를 사용하여 이를 동작하지 않게 하고 onClick에 넘겨준 것만 실행할 수 있게 해줍니다.
function clickEventFunc(e){ e.preventDefault(); console.log('페이지 이동 없이 클릭'); }
event를 인자로 받아 e.preventDefault()
를 호출하면, 이벤트의 기본동작은 동작하지 않게 되고 console.log
만 수행됩니다.
# function 바인딩 - bind()
기본적으로 onClick
등으로 전달한 이벤트에서 this
를 호출한 경우 바인딩 되어있지 않기 때문에 undefined
로 표시됩니다.
this는 render()함수가 포함되어 있는 컴포넌트 자체를 가르키는데 그 안에있는 function 내부에서 this는 바인딩을 해주지 않으면 아무값도 가지지 않습니다. 이러한 이유로 this를 바인딩 해 주어야 합니다.
아래와 같이 바인딩을 해주지 않으면 this의 state를 바꾸기 위해 this.setState
를 하는데 undefined
오류가 발생합니다.
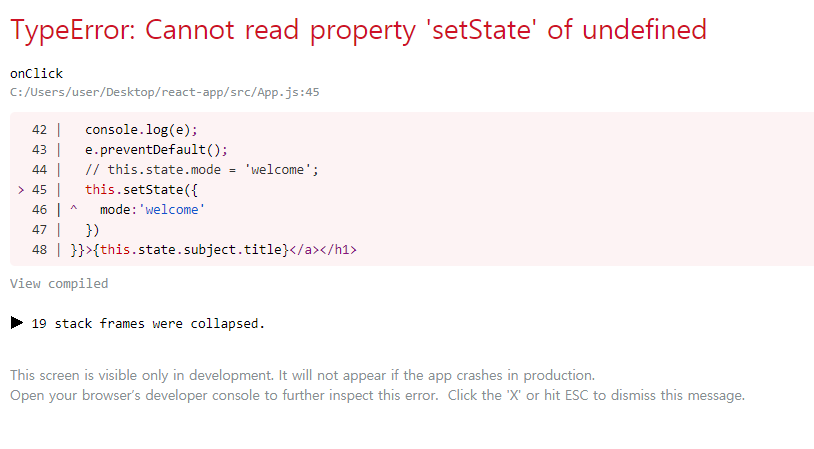
아래와 같이 해당 function
에 .bind(this)
로 this를 바인딩 시켜줍니다.
<h1> <a href="/" onClick={function(e){ console.log(e); e.preventDefault(); // this.state.mode = 'welcome'; this.setState({ mode:'welcome' }) }.bind(this)}>{this.state.subject.title}</a> // 해당 function에 .bind(this)로 this를 바인딩 시켜준다. </h1>
React 리액트 컴포넌트 이벤트 처리 기본예제 1
아래 예제는 이해를 돕기 위해 function을 따로 빼지 않고 onClick={function(e){...}.bind(this)} 로 직접 처리하였습니다.
App.js
- 부모 컴포넌트
import React, { Component } from 'react'; import TOC from "./components/TOC" import Content from "./components/Content" import Subject from "./components/Subject" import './App.css'; class App extends Component{ // 컴포넌트가 실행될 때 constructor가 먼저 실행되면서 초기화를 담당한다. constructor(props){ super(props); this.state ={ mode:'read', subject:{title:'WEB', sub:"World Wide Web!"}, welcome:{title:'Welcome', desc:'Hello, React!!'}, contents:[ {id:1, title:'HTML', desc:"HTML is for information"}, {id:2, title:'CSS', desc:"CSS is for design"}, {id:3, title:'JavaScript', desc:"JavaScript is for interactive"} ] } } render(){ // 렌더링이 될 때마다 state의 mode값을 확인하여 이벤트 처리 console.log('App render'); var _title, _desc = null; if(this.state.mode === 'welcome'){ _title = this.state.welcome.title; _desc = this.state.welcome.desc; }else if(this.state.mode === 'read'){ _title = this.state.contents[0].title; _desc = this.state.contents[0].desc; } return ( <div className="App"> {/* <Subject title={this.state.subject.title} sub={this.state.subject.sub}> </Subject> */} <header> <h1><a href="/" onClick={function(e){ console.log(e); e.preventDefault(); // a 태그의 기본 동작은 href로 페이지 이동이기 때문에 이동을 막기 위해 사용 // this.state.mode = 'welcome'; // 이렇게 하면 react는 state가 바꼈다는걸 인식하지 못하기 때문에 setState를 사용합니다. this.setState({ // 클릭 시 state의 mode의 값을 'welcome'으로 바꿉니다. mode:'welcome' }) }.bind(this)}>{this.state.subject.title}</a></h1> // function에서 this는 바인딩되어있지 않기 때문에 bind(this)를 통해 this를 바인딩 {this.state.subject.sub} </header> <TOC data={this.state.contents}></TOC> <Content title={_title} desc={_desc}></Content> // 렌더링 될 때 정의된 값을 넣어줍니다. </div> ); } } export default App;
Content.js
- 자식 컴포넌트
import React, { Component } from 'react'; class Content extends Component{ render(){ console.log('Content render'); return( <article> <h2>{this.props.title}</h2> {this.props.desc} </article> ); } } export default Content;
해서 이벤트에 따라 props의 던지는 값이 다르기 때문에 Content 컴포넌트도 이벤트에 따라 렌더링이 다시 되면서 props 값에 따라 화면을 다시 그리게 됩니다.
브라우저 화면
[WEB] 버튼 클릭 전
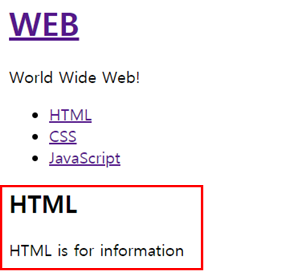
[WEB] 버튼 클릭 후
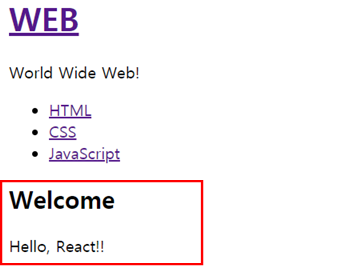
React 리액트 컴포넌트 이벤트 처리 기본예제 2
이번에는 자식 컴포넌트를 직접 호출하여 사용할 때 이벤트 처리 예제입니다.
App.js
- 부모 컴포넌트
return ( <div className="App"> <Subject title={this.state.subject.title} sub={this.state.subject.sub} // 이벤트 함수 작성 onChangePage = {function(){ this.setState({mode:'welcome'}); }.bind(this)} > </Subject> <TOC data={this.state.contents}></TOC> <Content title={_title} desc={_desc}></Content> </div> );
Subject 안에 onChangePage
라는 이벤트를 작성하고 자식 컴포넌트 쪽에서 이벤트를 호출 하는 형식으로 코드를 작성합니다. 이제 컴포넌트 사용자가 설치한 onChagePage 함수를 호출해 주기만 하면 됩니다.
onChangePage
함수는 어떠한 형태로 Subject.js
에 전달될까요? 바로 props
로 전달되겠죠?
Subject.js
- 자식 컴포넌트
import React, { Component } from 'react'; class Subject extends Component{ render(){ console.log('Subject render'); return( <header> <h1><a href="/" onClick={function(e){ // a 태그가 onClick될 때 e.preventDefault(); // a태그의 기본 작동인 href 이동 막음 this.props.onChangePage(); // props로 받아온 onChagePage()함수 호출 }.bind(this)}>{this.props.title}</a></h1> {this.props.sub} </header> ); } } export default Subject;
브라우저 화면
[WEB] 버튼 클릭 전
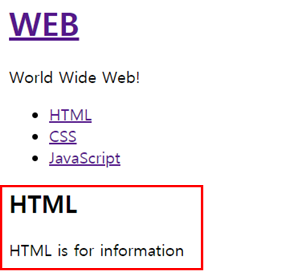
[WEB] 버튼 클릭 후
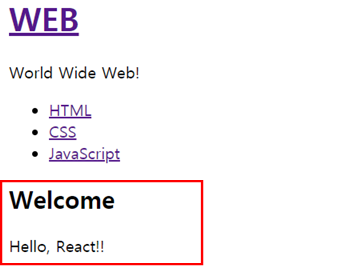
리액트 글 모두 보러 가기
devmoony.tistory.com/category/Web/React%20%EB%A6%AC%EC%95%A1%ED%8A%B8
'Web/React 리액트' 카테고리의 글 목록
devmoony.tistory.com
'Programming > React' 카테고리의 다른 글
[React 리액트] setState() - state 값 동적 변경 (0) | 2021.04.09 |
---|---|
[React 리액트] bind() 함수 이해하기 (개념 및 사용법) (0) | 2021.04.08 |
[React 리액트] Each child in a list shoul have a unipue "key" prop 오류 해결 방법 (0) | 2021.04.07 |
[React 리액트] 리스트(배열) 데이터 여러 개 출력하는 방법 (props, key) (0) | 2021.04.01 |
[React 리액트] props와 state 개념 및 기본 예제 (0) | 2021.03.31 |